Specifications that use this resource:
Teaching guide: areas of concern
The topics covered in our AS and A-level Computer Science specification are wide ranging in their scope. This document covers a few of the trickier topics offering tips and suggestions on how they can be delivered to students.
Functional programming and recursion
The functional programming paradigm is almost certainly a new topic for students and may be new to teachers. Functional programs aim to avoid side-effects by not using local, global or static variables, this means that each block of code within a functional program can be executed without it affecting other blocks of code.
The behaviour of a functional program should not be determined by the history of execution of the rest of the program, only by the inputs to that particular function.
Functional programming makes use of recursive techniques, a topic that students often struggle with. Functional programs tend to avoid side effects, so the programs blocks can be independently tested and the execution of programs can be highly optimised, running on multiple processor cores at once.
How to teach it
Teachers could use a range of languages to introduce functional programming, including languages their students may already be familiar with: C#, Python, Java. Familiarity with this paradigm might take a little getting used to and it might be a good opportunity to introduce a new language such as Haskell or Lisp which some people find more suited to teaching the subject. Exam questions will use Haskell notation.
When dealing with list programming, students may benefit from having physical representations to work with through the use of numbered or alphabetised cards, where they can split the head from the tail. They can then work through coded examples of functional programming. List functions are a good place to introduce the idea of recursion, for example repeatedly removing the head of a list and checking the new head, until you find the item you are looking for. This is an example of a linear search.
Having physical dry wipe cards which students can write on may also help students understand the map, filter and reduce/fold instructions.
Assembly code
Assembly code is a low-level programming language where one line of assembly code normally maps to one line of machine code (the 1s and 0s executed by the computer). Students may have experience using assembly code at KS4 or KS3, but care must be taken over which assembly language they used, with additional functionality and differences in instruction sets noted.
There are many different assembly languages available such as those specifically used to program certain processors and those created specifically for teaching (such as versions of the Little Man Computer). The language used for AQA’s examinations is based on the ARM Assembly Language used by the Raspberry Pi.
Several instructions not found in common implementations of the Little Man Computer are used by ARM Assembly Language:
Operation | Description |
---|---|
AND Rd, Rn, <operand2> | Perform a bitwise logical AND operation between the value in register n and the value specified by <operand2> and store the result in register d. |
ORR Rd, Rn, <operand2> | Perform a bitwise logical OR operation between the value in register n and the value specified by <operand2> and store the result in register d. |
EOR Rd, Rn, <operand2> | Perform a bitwise logical exclusive or (XOR) operation between the value in register n and the value specified by <operand2>and store the result in register d. |
MVN Rd, <operand2> | Perform a bitwise logical NOT operation on the value specified by <operand2> and store the result in register d. |
LSL Rd, Rn, <operand2> | Logically shift left the value stored in register n by the number of bits specified by <operand2> and store the result in register d. |
LSR Rd, Rn, <operand2> | Logically shift right the value stored in register n by the number of bits specified by <operand2> and store the result in register d. |
In the exam, students will be provided with a list of assembly language instructions so they will not need to recall the instruction set.
How to teach it
Depending on the time you have to dedicate to teaching Assembly code, you might choose to introduce it through the Little Man Computer emulator. Once students are familiar with the basic instructions you can then switch to a more complete Raspberry Pi implementation or an ARM processor emulator.
It is important that students have opportunities to write and debug assembly code, ideally through the use of a computer. Where you are unable to get the hardware/software for students to do this using a computer, getting students to write assembly code instructions on paper to solve given problems and handing it to their peers to trace and debug, can provide a suitable learning environment.
Object-orientation
The Object-orientated paradigm is one of the most important programming paradigms for modern computing. Students may have met it at lower key stages in the form of Greenfoot (Java), Python, C# etc. It is important to remember that the class is the description of an object and object is an instance of a class:
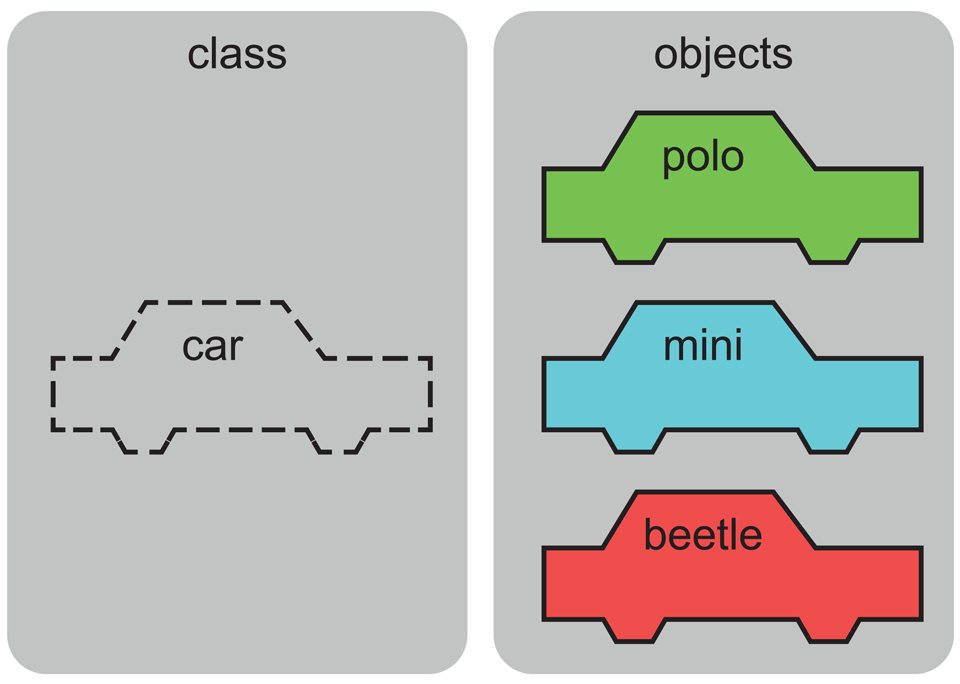
The fundamental encapsulated components of classes are methods (things that objects do) and attributes (information stored about objects).
- Association – Object B is linked to Object A, but A does not own B
- Composition – Object B is part of Object A, when A is destroyed, so is B
All the languages supported by AQA’s specifications allow students to write object-orientated code. However, there are differences in the ways that the languages implement object orientation, for example Java forces the use of objects for the most basic of programs and Python doesn’t allow the user to specify public, protected and private methods and attributes. When preparing your lessons make sure that you are familiar with the ways that your chosen programming language can be used to highlight the concepts on the course.
How to teach it
When starting to teach Object-orientation you can ground the topic in student experiences of the real world and computer games. For example you can show the students that they already use the object and class distinction in their everyday lives through naming types of things (classes) and giving examples of them (objects): this might be
student=class,
object=James, object=Syeda, object=Kimberley
Students can then come up with their own examples and start to list the attributes and methods needed for each class/object. Introductory object-orientated programming can involve students creating simple computer games with character classes defining their attributes and methods, instantiating them into objects and interacting with them. For example:
code:
dim Elf1 as new Elf('Sheila')
Elf1.eat()
Elf1.run()
output:
New Elf created with name Sheila
Sheila’s health = 110
Sheila’s strength = 90
Once the fundamentals are established you can look at the concept of inheritance through taxonomies. For example with our Elf code above, you might find that Elves and Wizards share very similar attributes and methods, meaning they can both inherit a parent class of 'character'.
Trees and treasure in the game also share attributes and methods and can inherit these from a parent class, 'assets'. Students can then draw out their own simple class diagrams:
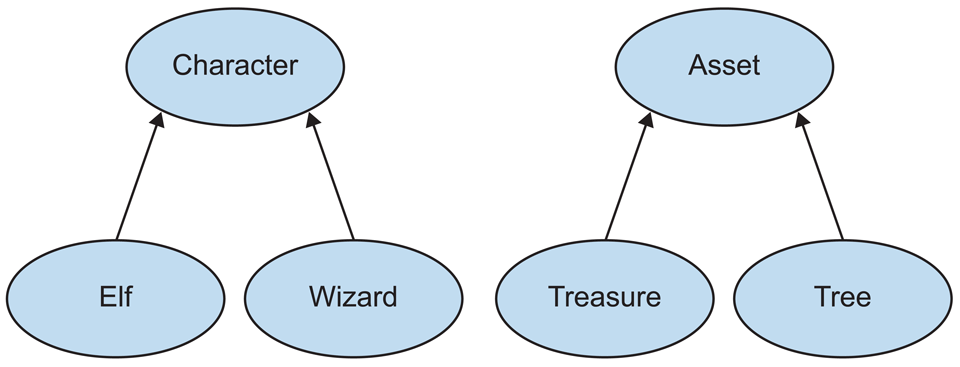
The attributes and methods within each class will be public, private and protected. These different access modifiers restrict how the methods and attributes are accessed by the class, any subclasses and the main program code.
Can the methods and attributes of a class be accessed by the:
Modifier | Class | Subclass | World code |
---|---|---|---|
Public | Y | Y | Y |
Protected | Y | Y | N |
Private | Y | N | N |
For example, the name of the character might be set when it is instantiated and there might be no need to change it again for the duration of the game, the subclasses Elf and Wizard would also like to use this method, so we would set it as Protected. For a method such as eat(), we want to be able to make the characters eat() due to external world events, such as walking over a sandwich asset, therefore we need to make this Public.
Further concepts of overriding and polymorphism can then be built into this computer game model, for example the class character could have an attack method, the functionality of which would change for the Elf and the Wizard.
Finally, you could introduce the idea of Aggregation by getting the Elf to either pick up a weapon that can then be put down (Association), or choose a weapon when an Elf is instantiated, the weapon will be destroyed when the Elf dies (Composition).
JSON and XML
Students may be familiar with storing data in databases, many websites and computer programs use JavaScript Object Notation (JSON) and EXtensible Markup Language (XML) as a simple and quick way to store data. JSON is considered by many to be a better and slicker way of storing information.
How to teach it
Students may have some experience of HTML tags which are close in functionality to how XML works. This could be extended to teaching Vector Graphics through Structured Vector Graphics (SVG), an image format that used XML. Programs such as Inkscape allow you to look at the XML created when designing images.
AQA’s A-level specification requires students to be able to compare and contrast the two formats. Giving students a task to use XML and JSON to encode a given data set will allow them to evaluate the effectiveness of both systems.
If you have more time available then Javascript can be used to read, create and manipulate XML and JSON data files. W3Schools offers a range of tutorials and tests to support you in this through their lessons on JSON and XML DOM. You can also see how JavaScript and XML can be combined to modify Google Maps.
Pointers
Pointers are fundamental programming constructs that allow a program to reference a given position in computer memory using its address. Pointers are used to create data types such as linked lists. All programs use pointers though many modern computer languages make it difficult to address memory locations explicitly with pointers. Languages such as C# and Pascal allow for the use of explicit pointer data types, whilst in Java and VB.Net it is very difficult to recreate this functionality.
How to teach it
In the additional information section of the specification it states that, 'Not all languages support explicit pointer types, but students should have an opportunity to understand this data type'. Pointers can be simulated in code using an array to implement a dynamic data type such as a linked list or a stack, and treating references to array locations as pseudo pointers. Students can also be asked to draw implementations of how pointers would be used to implement dynamic data structures such a circular queues.
If possible, and depending on the language, students could acquire experience with using pointers in a programming language.
Algorithms and complexity
The AS and A-level specifications outline the need for students to be familiar with a range of algorithms :
- Encryption
- Caesar Cipher
- Vernam Cipher
- Path finding (A-level only)
- Dijkstra’s shortest path algorithm
- Sorting
- Bubble Sort (A-level only)
- Merge Sort (A-level only)
- Searching
- Linear search (A-level only)
- Binary search (A-level only)
Students will be expected to understand these algorithms for the exams and they might also choose to implement them as part of their A-level non-exam assessed work (project).
In the case of Sorting and Searching, students should be aware of the time complexity of different algorithms. Time complexity doesn’t mean the number of seconds an algorithm takes to run, as the data could be such that running it the first time takes two seconds and running it another time takes two hours; it means the category of solution that an algorithm comes under.
For example the binary search is logarithmically faster than linear search. With linear search, in the worst case scenario that you were looking for an item in an array of length n, you would have to search each item from the first to the last until you found it (or found it wasn’t there). We can therefore describe linear search as having a time complexity O(n), where n means that the worst case scenario needs us to check every item in an n length input.
Binary search deals with ordered arrays, so it only need to pick the middle item and keep splitting the array until it finds the item it is looking for. The worst case scenario here would be log2n searches until you found the item (or found it wasn’t there). We can describe the nature of this algorithm as O(log2n).
Students should be able to:
Notation | Name | Examples |
---|---|---|
O(1) | constant | printing a value |
O(log n) | logarithmic | binary search |
O(n) | linear | linear search |
O(n2) | quadratic | bubble sort and the original version of Dijkstra’s Algorithm |
O(n3), O(n4), etc | polynomial | |
O(cn) | exponential | brute force password cracking |
O(n!) | factorial |
How to teach it
It is important that students understand how the algorithms above work.
For the searching and sorting, asking students to act out algorithms using cards, names and weights provides a perfect opportunity for students to learn the techniques and demonstrate that they understand them. From these demonstrations you might ask the students to explain the steps in pseudo code, then convert this to executable code.
For encryption students might act out sending secret message across the room, attempting to intercept and decrypt messages. With the Caesar cipher it is perfectly possible for students to crack the encryption technique. From these demonstrations you might ask the students to explain the steps in pseudo code, then convert this to an executable program. For the Caesar cipher students might write a brute force cracking program to attempt all possible encryption keys and find the original message.
For Dijkstra’s shortest path algorithm students might want to follow the steps on squared paper with various obstacles, acting out the process used by the algorithm.
In all the algorithms above students should have access to coded examples of each and practice tracing the steps on paper.
Data definition language (DDL)
Students may be used to defining the structure of databases using a graphical user interface such as the one found in Microsoft Access. DDL allows you to define and update the structure and relationships between database tables. For the non-exam assessment work (project), it is recommended that students use DDL if they are creating databases.
Care should be taken as different databases may use different key words, such as the datatype for a Boolean in Microsoft Access is ‘YESNO’
How to teach it
Students should first plan out what will be the structure of their database, listing tables names, field names, field types, size restrictions and relationships between tables.
A good place to get started is for students to complete some online tutorials using SQLZoo. Students can then start to construct statements to create their tables using the DDL knowledge they have learnt and their earlier table designs. DDL can be directly executed in Microsoft Access and debugging can occur here. Alternatively a package such as MySQL could be used.